How to count the number of values found for a field in MongoDB using Node.js and Mongoose (using aggregation)
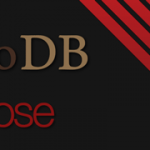
This post is part 1 of a series of posts I will make regarding Node.js and MongoDB. I will be using the Mongoose module for Node.js, but for the most part the MongoDB syntax is about the same. The ultimate goal with this series is to take data from a MongoDB database, and use that data to create a dynamic graph/chart with Morris.js.
The first step to this process is learning about MongoDB aggregation. The data we will be using in this example is a collection of products, that has a field product_manufacturer. We will use MongoDB to return to us, the total number of products that each manufacturer has in our database. So let’s get started ..
Sample MongoDB Schema/Data
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
{ "product_number": 1, "product_manufacturer": "Sony", } { "product_number": 2, "product_manufacturer": "LG", } { "product_number": 3, "product_manufacturer": "LG", } { "product_number": 4, "product_manufacturer": "Samsung", } { "product_number": 5, "product_manufacturer": "Samsung", } { "product_number": 5, "product_manufacturer": "Samsung", } |
So as you can see above we have 6 total products, 1 is Sony, 2 are LG, and 3 of them are Samsung. Now let’s use MongoDB’s aggregation framework and have it return to us the number of products for each manufacturer.
Writing the code
Using aggregation we will tell MongoDB (mongoose for node.js) to use our existing model and return to us a new collection with new fields containing the results.
1 2 3 4 5 6 7 8 9 |
ProductModel.aggregate( { $group: { _id: '$product_manufacturer', total_products: { $sum: 1 } } }, function (err, res) { if (err) return handleError(err); console.log(res); } ); |
The best way to think of how this works, mongoose will go through all documents in the ProductModel collection, it will check the product_manufacturer field and for each unique value (manufacturer), create a new document with a total_products field that is the total amount that were found.
The results
Here’s what the output would look like in console:
1 2 3 |
[ { _id: 'Sony', total_products: 1 }, { _id: 'LG', total_products: 2 }, { _id: 'Samsung', total_products: 3 } ] |
Amazing how easy that was, huh? For some reason this got very confusing for me and I spent a good day or two watching videos and reading online trying to figure out what needed to be done, and the answer ended up being so simple!
BUT … this is just the start of it, now that you understand the basic example of how to count the amount of products, we will next add some additional fields such as date_created, and then use MongoDB to only return products created between certain dates, etc. This is just the start, and in future parts of this series we will go over Arrays, and other ways to use aggregation provided by MongoDB.
Must watch videos regarding MongoDB Aggregation
The Aggregation Framework
This video will explain the entire MongoDB aggregation framework, how it works, and what options are available. You should definitely watch this video if you plan on using aggregation anytime in the near future.
MongoDB Training Course (m101 2 simple example)
This video is from the training courses that 10gen provides (for free, see below) and shows a very basic example of how we used aggregation above. This demo is specifically for MongoDB, but the only real difference is the example above using Mongoose has a callback, and doesn’t have the array ([]).
MongoDB University
MongoDB University is an online learning platform run by MongoDB available to anyone in the world with an internet connection. Their free courses will teach you how to develop for and administer MongoDB quickly and efficiently.
Frequent assessments help you verify your understanding, and at the end of a course, you’ll receive a certificate of completion from MongoDB. You’ll also become a member of MongoDB’s community of cutting-edge NoSQL technologists. Over time, they will be adding classes on schema design, advanced scaling and replication, and other topics.
MongoDB University’s platform was developed under a collaboration with edX, the not-for-profit consortium between MIT, Harvard and Berkeley.
So what are you waiting on, it’s free!
https://education.mongodb.com
-
Rahul Dabhi